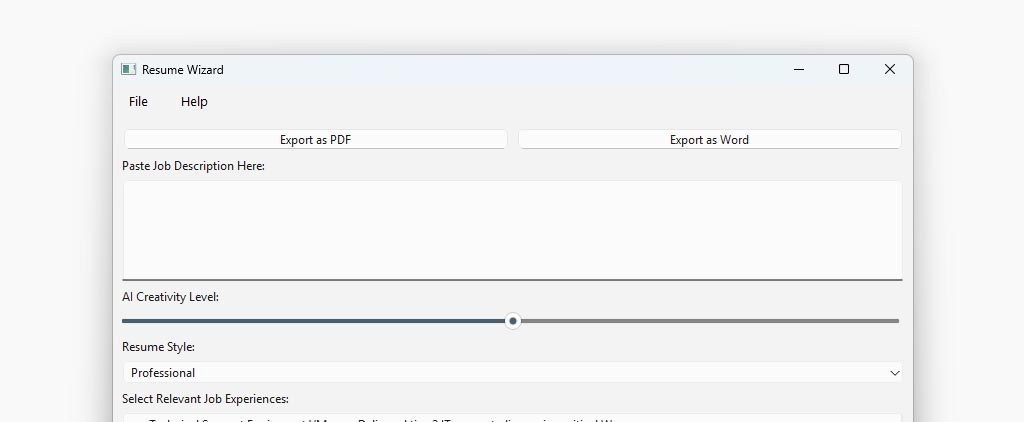
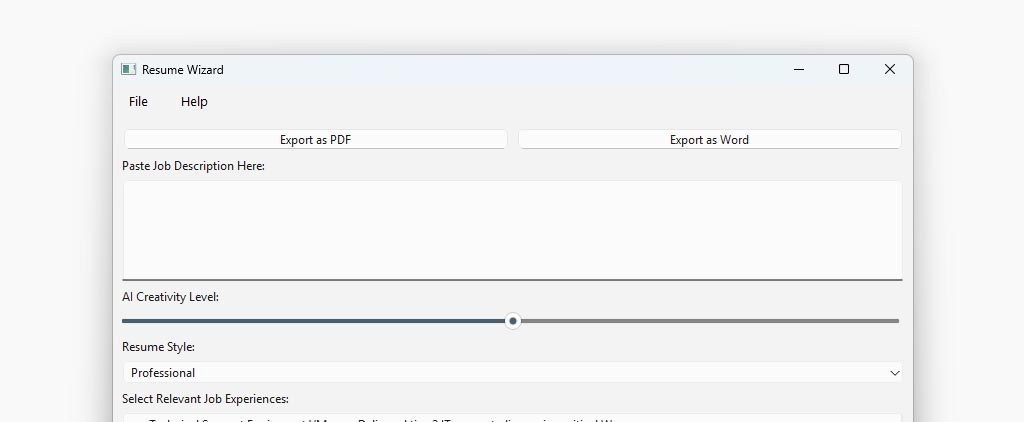
Tailoring my resume for each job application is one of the most time consuming parts of my job search. I carefully analyze job descriptions, compare the required skills to my own experience, and refine my resume to match. To streamline this process, I leverage ChatGPT to extract key keywords and seamlessly integrate them into a customized version of my resume.
Efficiency doesn’t stop at just improving my resume, I wanted to automate the entire process. So, I set out to build Resume Wizard, a Python based application that analyzes job descriptions, identifies critical skills, and generates an optimized resume using OpenAI’s API. With a bit of guidance, iteration, and well placed prompts, the code came together in a way that felt almost collaborative.
In this guide, I’ll take you through building, compiling, and publishing an AI-powered resume optimizer.
✅ Extracts skills from job descriptions using AI
✅ Matches past job experiences with the job requirements
✅ Generates tailored resumes in an editable format
✅ Exports resumes to PDF & Word (.docx)
✅ Fully functional standalone executable file
Ensure you have Python 3.9+ installed. Then, install the required libraries:
pip install PyQt6 openai python-docx reportlab dotenv
Description of libraries:
Create a project folder named resumeWizard
and organize it as follows:
resumeWizard/
│-- main.py # Main GUI Application
│-- ai_matching.py # OpenAI Job Description Analysis
│-- database.py # SQLite Database Functions
│-- api.env # Stores OpenAI API Key (Secret Key will NOT be committed to GitHub!!)
│-- requirements.txt # List of dependencies
│-- README.md # Project documentation
│-- assets/ # Screenshots for the blog post
│-- dist/ # Folder for the compiled executable
│-- .gitignore # Exclude sensitive files from GitHub
Complete project at my 🔗 GitHub Repository
main.py
):This is where we design the GUI using PyQt6 and integrate features such as job description analysis, experience selection, and resume preview.
🔹 Key Features Implemented:
Accepts a job description input.
Analyzes it using OpenAI to extract skills.
Displays relevant experiences from a database.
Exports the generated resume as a PDF.
ai_matching.py
):This script calls OpenAI API to extract relevant skills and generate an optimized resume.
ai_matching.py
) code on GitHubTo package the application as a standalone .exe
file, use PyInstaller and run this one line command below:
pip install pyinstaller python -m PyInstaller --noconfirm --onefile --windowed --icon=app.ico --hidden-import=PyQt6 --hidden-import=openai --add-data "api.env;." main.py
After compilation, find the .exe
in the dist/
folder.
This concludes the instructions and process I went through to build the Resume Wizard, an AI-powered resume optimizer. This Python based app allows you to generate tailored resumes in seconds. Feel free to check out the complete project on my GitHub.